How to Add a Search Bar in Table View
Step1
create a Project by using" single view application"
Step2
Add this code to ViewController.h file
//
// ViewController.h
// SearchBAr2
//
// Created by Dhanushka Adrian on 6/26/13.
// Copyright (c) 2013 Adrian. All rights reserved.
//
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController<UITabBarDelegate,UITableViewDataSource,UISearchBarDelegate>
{
IBOutlet UITableView *tableView;
IBOutlet UISearchBar *searchBAr;
NSArray *allItem;
NSMutableArray *displayItems;
}
@end
Step 3
add UISearchBar & UITableView
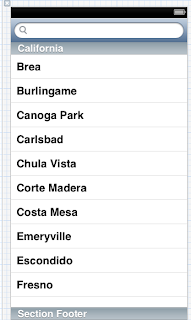
Step 4
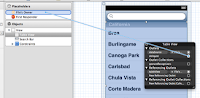
connect searchbar
Step 5
In ViewController change code like this
//
// ViewController.h
// SearchBAr2
//
// Created by Dhanushka Adrian on 6/26/13.
// Copyright (c) 2013 Adrian. All rights reserved.
//
#import "ViewController.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
allItem=[[NSArray alloc] initWithObjects:@"Supun",@"Adrian",@"Dhanushka",@"Kasun",@"Damith",@"Chamitha",@"Thilina",@"Asela",@"Shanika",@"Chandrakantha",@"Tharidu", nil];
displayItems=[[NSMutableArray alloc] initWithArray:allItem];
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyboardHidden:) name:UIKeyboardWillHideNotification object:nil];
// Do any additional setup after loading the view, typically from a nib.
}
//
-(NSInteger)numberOfSectionsInTableView:(UITableView *)tableView{
return 1;
}
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section{
return [displayItems count];
}
-(UITableViewCell *)tableView:(UITableView *)atableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//static NSString *simpleTableIdentifier = @"SimpleTableItem";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"cell"];
if (cell == nil) {
cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:@"cell"];
}
cell.textLabel.text = [displayItems objectAtIndex:indexPath.row];
return cell;
}
//when user type text in search bar ........(textChange)
-(void)searchBar:(UISearchBar *)searchBar textDidChange:(NSString *)searchText
{
if([searchText length] == 0)//if there nothig in search field
{
[displayItems removeAllObjects];
[displayItems addObjectsFromArray:allItem];
}
else
{
[displayItems removeAllObjects];
for(NSString *string in allItem)//every single item in allItem array
{
NSRange r=[string rangeOfString:searchText options:NSCaseInsensitiveSearch];//where it is
if(r.location!=NSNotFound)
{
[displayItems addObject:string];
}
}
}
[tableView reloadData];
}
-(void)keyboardHidden:(NSNotification *)note
{
[tableView reloadData];
}
-(void)keyboardShown:(NSNotification *)note{
CGRect keyboardFrame;
[[[note userInfo]objectForKey:UIKeyboardFrameEndUserInfoKey]getValue:&keyboardFrame];
}
//handle the search button click in keyboard------------
- (void)searchBarSearchButtonClicked:(UISearchBar *)asearchBar
{
[asearchBar resignFirstResponder];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
@end
Step 6
Run the App
Good Luck!
Tutorial List
- SQLite Based iPhone Application
- json with IOS (Basic) - Tutorial
- Simple Table View in IOS
- Load Distinct Images from array into UITableView
- Simple UIPickerView Example
- Tab Bar Application Part 1
- Tab Bar Application Part 2
- Tab Bar Application Part 3 ( with Login Screen )
- Tab Bar Application Part 4 ( Navigation )
- Table View Design in IOS Part 1
- Table View Design in IOS Part 2 ( Time Table )
- How to Add search bar in Table View
- Local Notification in IOS
- Core Data (For Beginners )
- Core Data Tute 2 (Add/Delete/Search)
- Core Data Tute 3 (Two table)
- Custom Cell in UITableview
- Lazy Loading
- Pull to Refresh in TableView
- Working with keyboard in Objective C - Part 1